Introducing a Custom 'map' Function in PowerShell for Functional Programming
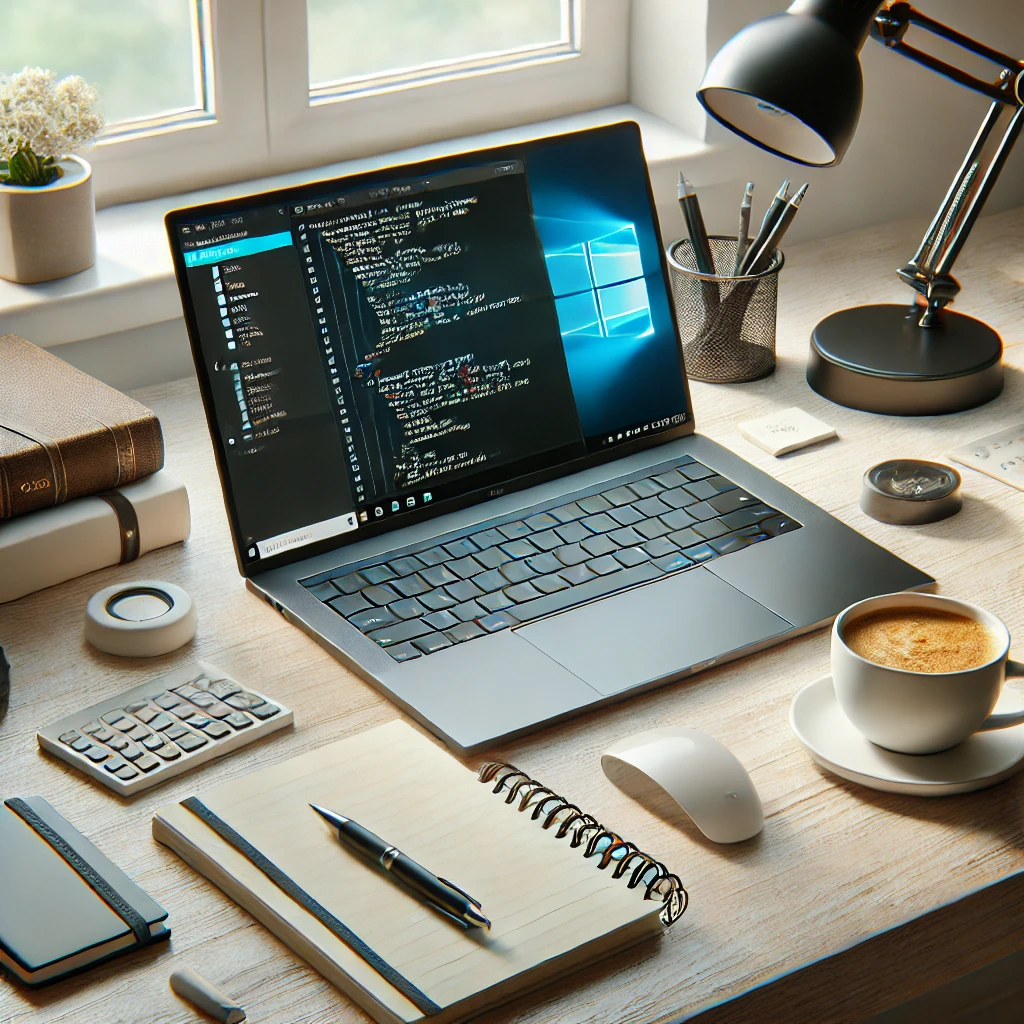
As developers, we’re always looking for ways to write cleaner, more efficient code. One of the paradigms that facilitate this is functional programming, which emphasizes the use of functions and immutability. While PowerShell is primarily an object-oriented scripting language, it also supports functional programming concepts. Recently, I implemented a custom map
function in PowerShell to process multiple arrays in a functional style. In this blog post, I’ll walk you through the implementation and show you how it can be used to simplify your data processing tasks.
The Need for a ‘map’ Function in PowerShell
In languages like Python and JavaScript, the map
function is a staple for transforming data collections. It applies a given function to each item of a list and returns a list of the results. While PowerShell has cmdlets like ForEach-Object
, they don’t natively support mapping over multiple arrays simultaneously. To bridge this gap, I created a custom map
function that can apply a function to multiple arrays in parallel.
The Implementation
Here’s the core of the map
function:
function map {
param(
$func,
[Parameter(ValueFromRemainingArguments = $true)]
$arrays
)
# Ensure all arrays are of the same length
$arrays | ForEach-Object {
if ($arrays[0].Length -ne $_.Length) {
throw "All arrays must be of the same length"
}
}
$dataType = $arrays.GetType().Name
if ($dataType -eq "Object[]") {
# If arrays are simple objects, apply the function directly
$arrays | ForEach-Object {
& $func $_
}
}
elseif ($dataType -eq 'List`1') {
# If arrays are lists, process them in parallel
$arrayCount = $arrays.Count
$count = $arrays[0].Length
# Get the parameter names of the function
if ($func -is [scriptblock]) {
$funcParams = $func.Ast.ParamBlock.Parameters | ForEach-Object { $_.Name.VariablePath.UserPath }
}
else {
$funcParams = (Get-Command $func).Parameters.Keys -as [array]
}
# Iterate over each index and apply the function
for ($i = 0; $i -lt $count; $i++) {
$funcSplat = [ordered]@{}
for ($ac = 0; $ac -lt $arrayCount; $ac++) {
$funcSplat["$($funcParams[$ac])"] = $arrays[$ac][$i]
}
& $func @funcSplat
}
}
}
Key Features
- Parallel Processing: The function can take multiple arrays and apply a user-defined function to corresponding elements.
- Dynamic Parameter Handling: It extracts parameter names from the provided script block or function, allowing for flexible argument passing.
- Type Handling: It checks the data type of the input to ensure proper processing, distinguishing between simple arrays and lists.
Usage Example
Let’s see how this function works with a practical example. Suppose we have three arrays containing names, ages, and locations:
$who = 'John', 'Jane', 'Doe', 'Smith'
$age = 20, 30, 40, 50
$where = 'New York', 'California', 'Texas', 'Florida'
We can use the map
function to combine these arrays into custom objects:
map {
param($who, $age, $where)
[PSCustomObject][Ordered]@{
Name = $who
Age = $age
Location = $where
}
} $who $age $where
Output:
Name Age Location
---- --- --------
John 20 New York
Jane 30 California
Doe 40 Texas
Smith 50 Florida
The map
function applies the script block to each set of elements from the arrays ($who[$i]
, $age[$i]
, $where[$i]
) and constructs a custom object with the combined data.
How It Works
- Parameter Extraction: The function retrieves the parameter names from the provided script block using the Abstract Syntax Tree (AST).
- Length Verification: It checks that all input arrays are of the same length to avoid index out-of-range errors.
- Function Invocation: It uses a loop to iterate over the index of the arrays and splats the parameters into the function for each iteration.
Benefits
- Enhanced Readability: By abstracting the iteration logic, the
map
function allows you to focus on the transformation logic. - Reusability: You can use the
map
function with any number of arrays and any processing logic encapsulated in a script block or function. - Functional Programming Style: This approach promotes immutability and pure functions, which can lead to fewer side effects and easier debugging.
Conclusion
The custom map
function brings the power of functional programming’s map operation to PowerShell, enabling parallel processing of multiple arrays with ease. By leveraging script blocks and parameter extraction, it provides a flexible tool for data transformation tasks.
If you often find yourself processing multiple related arrays, consider adding this map
function to your toolkit. It can simplify your scripts and make your code more expressive and maintainable.
Happy scripting!